Pacing response#
using ModelingToolkit
using OrdinaryDiffEq, SteadyStateDiffEq, DiffEqCallbacks
using Plots
using CSV
using DataFrames
using CaMKIIModel
using CaMKIIModel: second
Plots.default(lw=1.5)
Setup the ODE system#
Electrical stimulation starts at t
=100 seconds and ends at t
=300 seconds.
sys = build_neonatal_ecc_sys(simplify=true, reduce_iso=true, reduce_camk=true)
tend = 500.0second
prob = ODEProblem(sys, [], tend)
stimstart = 100.0second
stimend = 300.0second
@unpack Istim = sys
alg = KenCarp47()
KenCarp47(; linsolve = nothing, nlsolve = OrdinaryDiffEqNonlinearSolve.NLNewton{Rational{Int64}, Rational{Int64}, Rational{Int64}, Nothing}(1//100, 10, 1//5, 1//5, false, true, nothing), precs = DEFAULT_PRECS, smooth_est = true, extrapolant = linear, controller = PI, autodiff = ADTypes.AutoForwardDiff(),)
Single pulse#
callback = build_stim_callbacks(Istim, stimstart + 1second; period=10second, starttime=stimstart)
@time sol = solve(prob, alg; callback)
9.131137 seconds (18.51 M allocations: 885.086 MiB, 3.10% gc time, 99.44% compilation time)
retcode: Success
Interpolation: 3rd order Hermite
t: 132-element Vector{Float64}:
0.0
0.019333123284849308
0.09098129546757994
0.20735582943983855
0.45611910276862533
1.0472562928406122
2.0342667657223696
3.451776641148454
6.733792423912806
12.450117081023194
⋮
230382.26789998438
250334.35482912764
276733.2389256879
305590.06370566715
343648.8493253473
381707.6349450274
426138.04698145017
478964.361341805
500000.0
u: 132-element Vector{Vector{Float64}}:
[150952.75035000002, 13838.37602, -68.79268, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113 … 0.26081, 0.00977, 0.00188, 0.09243, 0.22156, 0.966, 0.00702, 830.0, 830.0, 0.0026]
[150952.7504077194, 13838.375711519551, -68.79732946186226, 0.1211319185255175, 0.1211301421535992, 0.12113000811850358, 0.12113000037367234, 0.12113000001389383, 0.12113000000038378, 0.12113000000000405 … 0.2608055799112056, 0.009769882842069916, 0.001879826176081169, 0.09242766669618695, 0.2215639938807744, 0.9660018276402699, 0.007020208561323646, 829.9999654017583, 829.9994975880693, 0.0025985659590295374]
[150952.75062244057, 13838.374513299192, -68.81607005265552, 0.12115248970210647, 0.12113624253259858, 0.12113143375871602, 0.12113027926811751, 0.12113004704056851, 0.12113000693481112, 0.12113000089551408 … 0.26078941009382306, 0.009769459049732313, 0.0018789042446514422, 0.09241901973901424, 0.2215787945110049, 0.9660085993318799, 0.00702008986027517, 829.9998348635061, 829.9976422525165, 0.002593310524681686]
[150952.7509740238, 13838.372555328333, -68.84681913285226, 0.12118951551963696, 0.12115723910142134, 0.12114109135582982, 0.12113403405679991, 0.12113131833063846, 0.12113038966346883, 0.12113010483012127 … 0.2607638707001999, 0.009768805322556195, 0.0018766436937193197, 0.09240497563401104, 0.22160283236040595, 0.9660195931792828, 0.007017234889558555, 829.9996133960318, 829.9946506157202, 0.0025849478335103777]
[150952.7517373371, 13838.368384399068, -68.91207316777846, 0.12125418995384041, 0.12120713813483534, 0.12117519662647316, 0.12115494411090462, 0.12114295934422187, 0.12113633873395535, 0.12113292110288301 … 0.2607122383588733, 0.00976754294689657, 0.0018699196262940568, 0.0923749578279845, 0.2216542075240971, 0.9660430731013478, 0.0070030143052343926, 829.9990964774663, 829.9883445519497, 0.00256770780104998]
[150952.75361533565, 13838.358559292059, -69.06429960994137, 0.12136450208851983, 0.1213063255235844, 0.12125925929399752, 0.12122229800810434, 0.12119414515426227, 0.1211733598588045, 0.12115849269665879 … 0.26060507831375035, 0.009765228548607825, 0.001850533769446942, 0.09230364339951613, 0.22177624785138977, 0.9660987611411453, 0.006946470397388757, 829.9976227528975, 829.9738229154025, 0.0025298083713907005]
[150952.75694915713, 13838.34240329337, -69.31024285744343, 0.1214919651325424, 0.12142927821043338, 0.12137426379919636, 0.12132672249013034, 0.12128628091507473, 0.12125242557826561, 0.12122454121844703 … 0.26047114911179464, 0.009763403076884485, 0.0018178174419014683, 0.09218462776986637, 0.2219798989175973, 0.9661914345949096, 0.0068337541728754475, 829.9944193668179, 829.9509077855928, 0.0024748079782158957]
[150952.76215620188, 13838.319723120678, -69.64637448767432, 0.12162015426876954, 0.12155743299711902, 0.12149988983034497, 0.1214475947100359, 0.12140052118634262, 0.12135855488207907, 0.12132150441596071 … 0.2603648729749554, 0.009765046552800477, 0.001773815690753224, 0.09201383579531924, 0.22227216398019892, 0.9663239343003682, 0.006675512062656749, 829.9883533251192, 829.9205247545176, 0.0024106893964811324]
[150952.77598869594, 13838.2694938995, -70.35063788657428, 0.12180447948880577, 0.12174768443532356, 0.12169340184085964, 0.12164180995533558, 0.12159304920072024, 0.12154722283384184, 0.12150439833798556 … 0.26041507667541997, 0.009786689200123853, 0.0016855899094922325, 0.09161908329520764, 0.22294828828976576, 0.9666286957812559, 0.006355039713677859, 829.9689941817725, 829.8589858006806, 0.002310655727734339]
[150952.80529264064, 13838.18882346682, -71.35304509818071, 0.12196378364408175, 0.12191937753328333, 0.12187579038290348, 0.1218331857334074, 0.12179171693004406, 0.12175152667167365, 0.12171274673876366 … 0.26104417804603025, 0.009873892913308327, 0.0015688568862426045, 0.09093422465365385, 0.22412560303273427, 0.9671554512230358, 0.005926150085676071, 829.923524981244, 829.7704141396295, 0.002228995040224517]
⋮
[151980.49074492313, 12814.832025762427, -68.85100718229718, 0.1276738374393192, 0.12767399755083778, 0.12767415863271986, 0.12767432073580456, 0.12767448391298603, 0.12767464821931834, 0.12767481371212686 … 0.0014250425920808707, 0.0017627343869550238, 0.0027285195506673056, 0.001895368871629723, 0.9996467050337319, 0.9996452040133846, 0.006998369446473842, 770.8455738105363, 770.6180101449373, 0.0013110574933418069]
[152030.0095482799, 12765.465796263097, -68.74145017349927, 0.12716703831660203, 0.1271671847887531, 0.12716733218593723, 0.12716748055541274, 0.12716762994634875, 0.12716778040992185, 0.12716793199941973 … 0.0014494175494680936, 0.0017771286009795836, 0.0027507932287871625, 0.0019253097273400721, 0.9996381496043464, 0.9996367238772486, 0.007050663608266816, 769.7602714710622, 769.5393751661346, 0.0012741355521194066]
[152089.0940693979, 12706.56321768799, -68.60863911197836, 0.12657564081673453, 0.1265757708263141, 0.12657590169462574, 0.12657603346437274, 0.12657616617998446, 0.12657629988770436, 0.1265764346356836 … 0.0014795265241537895, 0.0017947341209557058, 0.0027780353988237517, 0.0019622257232030154, 0.9996274985485912, 0.9996261714058949, 0.007114578819293557, 768.4520130329507, 768.2387727804979, 0.0012316600726947993]
[152146.18788140826, 12649.644845425024, -68.47806698979574, 0.12601823921093458, 0.1260183531694397, 0.12601846791140234, 0.1260185834748719, 0.12601869989943618, 0.12601881722629857, 0.12601893549836246 … 0.0015097502249171789, 0.0018122098061102874, 0.0028050767457020198, 0.0019991925634235182, 0.9996167185693637, 0.9996154980411869, 0.007177976172074255, 767.1767876740753, 766.970638765287, 0.0011922386884309721]
[152211.07834077455, 12584.95295851881, -68.3268845769729, 0.12540197835235595, 0.12540207398832906, 0.1254021703093507, 0.1254022673479053, 0.12540236513779035, 0.1254024637141832, 0.12540256311371234 … 0.0015455006404850298, 0.0018326547166288188, 0.0028367114583480795, 0.0020428447713242022, 0.9996038444026755, 0.9996027584322296, 0.007252083039670829, 765.7180271044275, 765.5195749729319, 0.0011493592919893181]
[152265.8434814776, 12530.354388202599, -68.19690157518868, 0.12489654993076008, 0.12489663008685117, 0.1248967108361876, 0.12489679220637957, 0.12489687422615271, 0.12489695692540577, 0.12489704033527076 … 0.001576913902917453, 0.0018504141852860735, 0.0028641907801204014, 0.0020811183826731417, 0.9995924290096039, 0.9995914681931636, 0.007316405055191646, 764.4825164865578, 764.2902625882477, 0.0011147544939316347]
[152319.0722897469, 12477.286289996282, -68.06838779298025, 0.12441852776173556, 0.12441859297044619, 0.12441865867612517, 0.12441872490152359, 0.12441879167031158, 0.12441885900712564, 0.12441892693761843 … 0.0016086228951629694, 0.0018681391558313628, 0.00289161645531835, 0.0021196471902841453, 0.9995808148786488, 0.9995799900737989, 0.007380556069262499, 763.2808158222887, 763.0943273877343, 0.0010825024540411798]
[152370.08344819857, 12426.427746933492, -67.94313901357017, 0.12397299209801999, 0.12397304313212638, 0.12397309456511595, 0.12397314641499346, 0.12397319870049137, 0.12397325144110685, 0.12397330465714136 … 0.0016401512461383411, 0.001885574798250941, 0.002918593728023189, 0.0021578658805081865, 0.9995691727691395, 0.9995684937018386, 0.0074436134182214436, 762.1313762816151, 761.9501759677468, 0.0010528653862354994]
[152387.287370467, 12409.274925456692, -67.9004151038974, 0.12382562805332914, 0.12382567443614112, 0.12382572118174298, 0.12382576830649855, 0.12382581582743342, 0.12382586376226862, 0.12382591212945655 … 0.0016509231088660363, 0.0018915560796425944, 0.00292785345451338, 0.00217106068478802, 0.9995651368709008, 0.9995644996549924, 0.0074652460137272425, 761.7447831893576, 761.5653135941376, 0.0010431528707010307]
plot(sol, idxs=(sys.t / 1000 - 100, sys.vm), title="Action potential (single pulse)", ylabel="mV", xlabel="Time (s)", label=false, tspan=(100second, 103second))
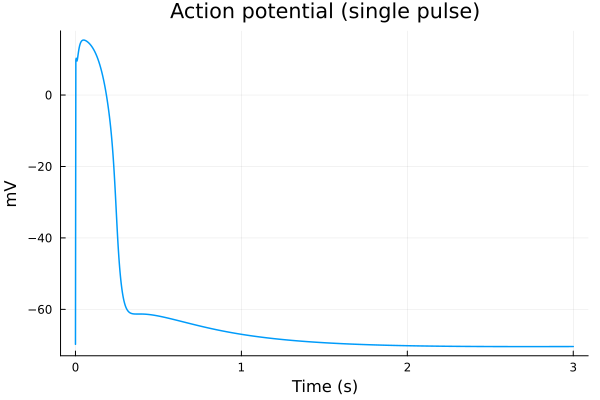
plot(sol, idxs=(sys.t / 1000 - 100, sys.Cai_mean), tspan=(100second, 103second), title="Calcium transient", ylabel="Conc. (μM)", xlabel="Time (s)", label="Avg Ca (Model)")
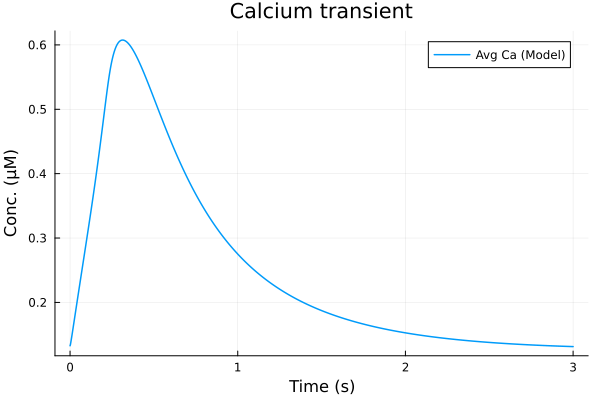
savefig("single-cat.pdf")
"/home/github/actions-runner-2/_work/camkii-cardiomyocyte-model/camkii-cardiomyocyte-model/.cache/docs/single-cat.pdf"
1Hz#
callback = build_stim_callbacks(Istim, stimend; period=1second, starttime=stimstart)
@time sol = solve(prob, alg; callback)
1.606767 seconds (78.93 k allocations: 21.183 MiB)
retcode: Success
Interpolation: 3rd order Hermite
t: 8928-element Vector{Float64}:
0.0
0.019333123284849308
0.09098129546757994
0.20735582943983855
0.45611910276862533
1.0472562928406122
2.0342667657223696
3.451776641148454
6.733792423912806
12.450117081023194
⋮
397420.7293508087
407496.2619864756
419304.0528694844
431111.8437524932
444428.3784047912
459536.4436840207
475039.8195722815
494235.3907788104
500000.0
u: 8928-element Vector{Vector{Float64}}:
[150952.75035000002, 13838.37602, -68.79268, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113 … 0.26081, 0.00977, 0.00188, 0.09243, 0.22156, 0.966, 0.00702, 830.0, 830.0, 0.0026]
[150952.7504077194, 13838.375711519551, -68.79732946186226, 0.1211319185255175, 0.1211301421535992, 0.12113000811850358, 0.12113000037367234, 0.12113000001389383, 0.12113000000038378, 0.12113000000000405 … 0.2608055799112056, 0.009769882842069916, 0.001879826176081169, 0.09242766669618695, 0.2215639938807744, 0.9660018276402699, 0.007020208561323646, 829.9999654017583, 829.9994975880693, 0.0025985659590295374]
[150952.75062244057, 13838.374513299192, -68.81607005265552, 0.12115248970210647, 0.12113624253259858, 0.12113143375871602, 0.12113027926811751, 0.12113004704056851, 0.12113000693481112, 0.12113000089551408 … 0.26078941009382306, 0.009769459049732313, 0.0018789042446514422, 0.09241901973901424, 0.2215787945110049, 0.9660085993318799, 0.00702008986027517, 829.9998348635061, 829.9976422525165, 0.002593310524681686]
[150952.7509740238, 13838.372555328333, -68.84681913285226, 0.12118951551963696, 0.12115723910142134, 0.12114109135582982, 0.12113403405679991, 0.12113131833063846, 0.12113038966346883, 0.12113010483012127 … 0.2607638707001999, 0.009768805322556195, 0.0018766436937193197, 0.09240497563401104, 0.22160283236040595, 0.9660195931792828, 0.007017234889558555, 829.9996133960318, 829.9946506157202, 0.0025849478335103777]
[150952.7517373371, 13838.368384399068, -68.91207316777846, 0.12125418995384041, 0.12120713813483534, 0.12117519662647316, 0.12115494411090462, 0.12114295934422187, 0.12113633873395535, 0.12113292110288301 … 0.2607122383588733, 0.00976754294689657, 0.0018699196262940568, 0.0923749578279845, 0.2216542075240971, 0.9660430731013478, 0.0070030143052343926, 829.9990964774663, 829.9883445519497, 0.00256770780104998]
[150952.75361533565, 13838.358559292059, -69.06429960994137, 0.12136450208851983, 0.1213063255235844, 0.12125925929399752, 0.12122229800810434, 0.12119414515426227, 0.1211733598588045, 0.12115849269665879 … 0.26060507831375035, 0.009765228548607825, 0.001850533769446942, 0.09230364339951613, 0.22177624785138977, 0.9660987611411453, 0.006946470397388757, 829.9976227528975, 829.9738229154025, 0.0025298083713907005]
[150952.75694915713, 13838.34240329337, -69.31024285744343, 0.1214919651325424, 0.12142927821043338, 0.12137426379919636, 0.12132672249013034, 0.12128628091507473, 0.12125242557826561, 0.12122454121844703 … 0.26047114911179464, 0.009763403076884485, 0.0018178174419014683, 0.09218462776986637, 0.2219798989175973, 0.9661914345949096, 0.0068337541728754475, 829.9944193668179, 829.9509077855928, 0.0024748079782158957]
[150952.76215620188, 13838.319723120678, -69.64637448767432, 0.12162015426876954, 0.12155743299711902, 0.12149988983034497, 0.1214475947100359, 0.12140052118634262, 0.12135855488207907, 0.12132150441596071 … 0.2603648729749554, 0.009765046552800477, 0.001773815690753224, 0.09201383579531924, 0.22227216398019892, 0.9663239343003682, 0.006675512062656749, 829.9883533251192, 829.9205247545176, 0.0024106893964811324]
[150952.77598869594, 13838.2694938995, -70.35063788657428, 0.12180447948880577, 0.12174768443532356, 0.12169340184085964, 0.12164180995533558, 0.12159304920072024, 0.12154722283384184, 0.12150439833798556 … 0.26041507667541997, 0.009786689200123853, 0.0016855899094922325, 0.09161908329520764, 0.22294828828976576, 0.9666286957812559, 0.006355039713677859, 829.9689941817725, 829.8589858006806, 0.002310655727734339]
[150952.80529264064, 13838.18882346682, -71.35304509818071, 0.12196378364408175, 0.12191937753328333, 0.12187579038290348, 0.1218331857334074, 0.12179171693004406, 0.12175152667167365, 0.12171274673876366 … 0.26104417804603025, 0.009873892913308327, 0.0015688568862426045, 0.09093422465365385, 0.22412560303273427, 0.9671554512230358, 0.005926150085676071, 829.923524981244, 829.7704141396295, 0.002228995040224517]
⋮
[151515.81902269452, 13278.08979441267, -69.80948561270954, 0.1328911379835353, 0.13289141451261458, 0.13289169194332418, 0.1328919703479174, 0.13289224980156938, 0.13289253038252608, 0.13289281217226254 … 0.0012285528850311776, 0.0016416151325810468, 0.002541088856024066, 0.001652105068639915, 0.9997133772334795, 0.9997114391130609, 0.006556983221575075, 780.2814530655273, 779.9799229341158, 0.0017175387418230242]
[151561.93420609168, 13232.111502348567, -69.71950082942882, 0.13233797446454454, 0.13233824058769578, 0.13233850766199565, 0.13233877575865619, 0.1323390449517689, 0.13233931531845164, 0.13233958693900466 … 0.0012457798340354403, 0.0016526273431767711, 0.0025581308492387835, 0.0016735787025274056, 0.9997076981393553, 0.9997057866510969, 0.006597217970035302, 779.4187091590055, 779.1254557091947, 0.001672274652495773]
[151613.37827166222, 13180.821155643242, -69.61787562491207, 0.1317297589157246, 0.1317300131317941, 0.13173026834243698, 0.13173052461740697, 0.13173078202927768, 0.13173104065358632, 0.13173130056898655 … 0.0012655289614995969, 0.001665150595298716, 0.0025775110554033497, 0.0016981584269493862, 0.9997011459431062, 0.9996992708969177, 0.006642946130775073, 778.4349956840823, 778.1507291507584, 0.0016230763240733606]
[151662.1597807227, 13132.186348571662, -69.5202549325179, 0.13116174360835592, 0.13116198617546715, 0.1311622297674355, 0.13116247445234583, 0.13116272030103537, 0.13116296738723435, 0.131163215787715 … 0.0012847925838740538, 0.0016772709472780449, 0.0025962676610781408, 0.0017221029092301628, 0.9996947156488464, 0.9996928738534642, 0.0066871791979826985, 777.4819598907359, 777.2059766533764, 0.0015776772056565387]
[151714.1718773969, 13080.331564596252, -69.41480081459333, 0.13056563831715426, 0.13056586814310067, 0.13056609901455363, 0.1305663309975021, 0.1305665641606037, 0.13056679857532064, 0.13056703431606428 … 0.0013059353333552443, 0.0016904606839283124, 0.002616678863411176, 0.0017483390578108323, 0.9996876101843762, 0.9996858116842525, 0.006735286219291705, 776.445184078569, 776.1777778843629, 0.001530612273689703]
[151769.5123137427, 13025.159389549324, -69.30099188927659, 0.1299423792731084, 0.12994259520758403, 0.12994281219687584, 0.1299430303044511, 0.12994324959634296, 0.12994347014128127, 0.12994369201083156 … 0.0013291436146088994, 0.0017048103584189416, 0.0026388847980878174, 0.0017770923131243625, 0.9996797565978022, 0.9996780071094088, 0.006787588903356696, 775.3199137735127, 775.0613455883323, 0.0014820507290665406]
[151822.53057122996, 12972.303078706258, -69.19037200428511, 0.12935606744648517, 0.12935626978484277, 0.12935647317406976, 0.1293566776748891, 0.1293568833504784, 0.12935709026659553, 0.12935729849171132 … 0.0013520978330366562, 0.001718871154455926, 0.002660643386483494, 0.0018054801341618916, 0.9996719302296265, 0.9996702372156245, 0.006838802266503445, 774.2215546069687, 773.9711768711558, 0.0014369811358477809]
[151883.26445998743, 12911.755414462972, -69.06164930516206, 0.1286976580943877, 0.12869784447431312, 0.1286980318860501, 0.1286982203868186, 0.12869841003615154, 0.12869860089601262, 0.12869879303092197 … 0.001379307402672207, 0.001735381405031326, 0.0026861928533384208, 0.0018390733838012786, 0.9996625831123113, 0.9996609568705979, 0.006898902681073257, 772.9407063683581, 772.6993808014286, 0.001387088933892788]
[151900.50607632753, 12894.566779686207, -69.02471233893911, 0.1285133581151902, 0.12851353988656045, 0.12851372268165784, 0.12851390655664113, 0.12851409156993898, 0.12851427778236563, 0.12851446525724405 … 0.001387219462961557, 0.001740147454442854, 0.0026935677621345395, 0.0018488280488430894, 0.999659852305361, 0.9996582450741127, 0.006916241631924592, 772.5729695511367, 772.3341498101114, 0.001373261522597776]
plot(sol, idxs=(sys.t / 1000, sys.vm), title="Action potential", ylabel="mV", xlabel="Time (s)", label=false)
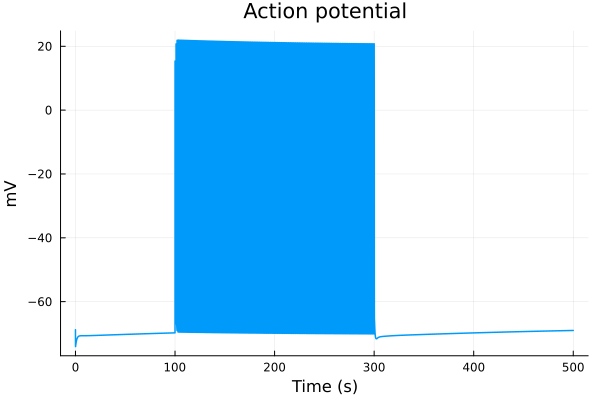
plot(sol, idxs=(sys.t / 1000 - 299, sys.vm), title="Action potential", tspan=(299second, 300second), ylabel="mV", xlabel="Time (s)", label=false)
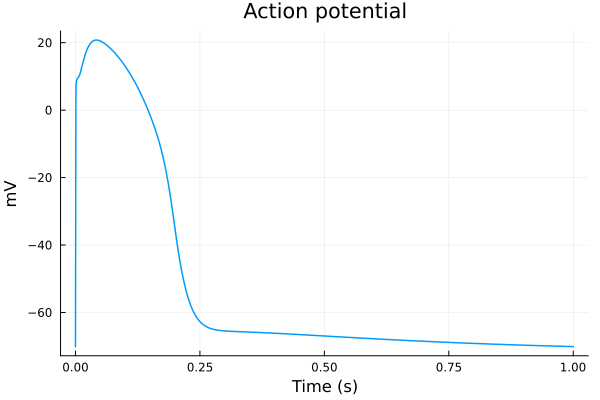
plot(sol, idxs=(sys.t / 1000 - 299, [sys.IK1, sys.Ito, sys.IKs, sys.IKr, sys.If]), tspan=(299second, 300second), ylabel="μA/μF", xlabel="Time (s)", label=["IK1" "Ito" "IKs" "IKr" "If"])
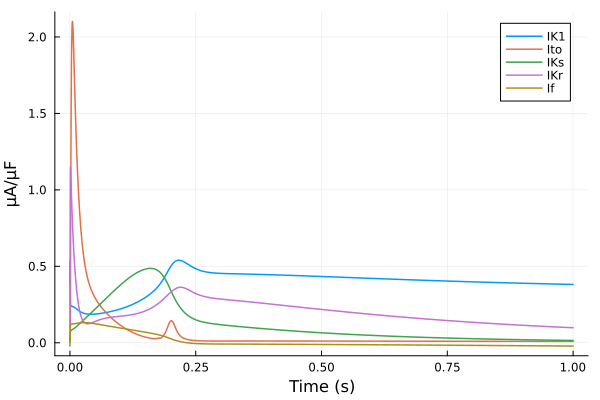
plot(sol, idxs=(sys.t / 1000 - 299, [sys.ICaL, sys.INaCa, sys.ICaT, sys.ICab]), tspan=(299second, 300second), ylabel="μA/μF", xlabel="Time (s)", label=["ICaL" "INaCa" "ICaT" "ICab"])
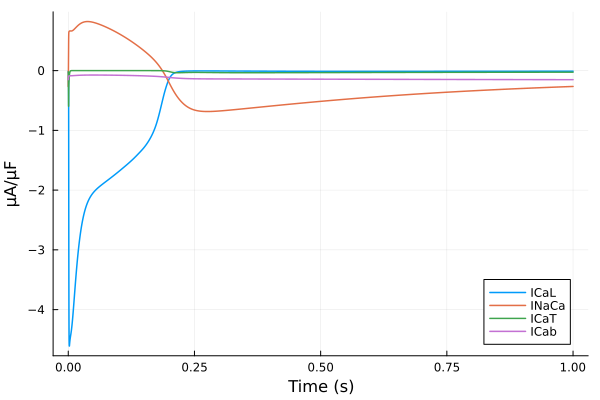
plot(sol, idxs=(sys.t / 1000 - 299, [sys.Cai_sub_SR, sys.Cai_sub_SL, sys.Cai_mean]), tspan=(299second, 300second), title="Calcium transient", ylabel="μM", xlabel="Time (s)", label=["CaSSR" "CaSL" "CaAvg"])
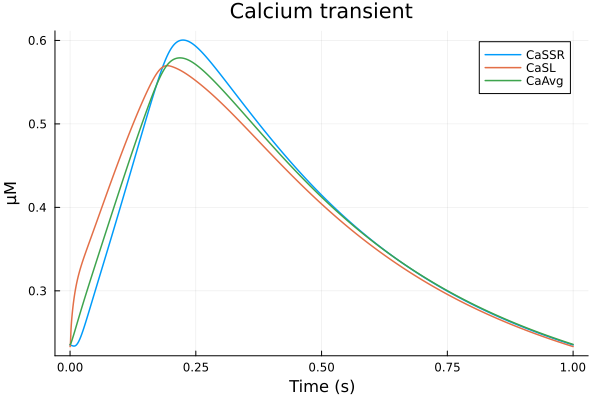
plot(sol, idxs=(sys.t / 1000, sys.CaMKAct * 100), title="Active CaMKII", ylabel="Active CaMKII (%)", xlabel="Time (s)", label=false)
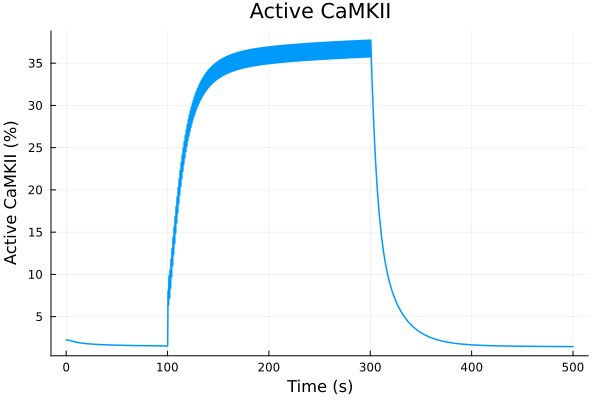
3D surface plot#
xx = 1:44
yy = range(299second, 300second, length=100)
zz = [sol(t, idxs=sys.Cai[u]) for t in yy, u in xx]
surface(xx, yy ./ 1000, zz, colorbar=:none, yguide="sec.", zguide="Conc. (μM)", xticks=false, size=(600, 600))
annotate!(3, 299, 0.65, "SL")
annotate!(41, 299.25, 0.58, "SR")
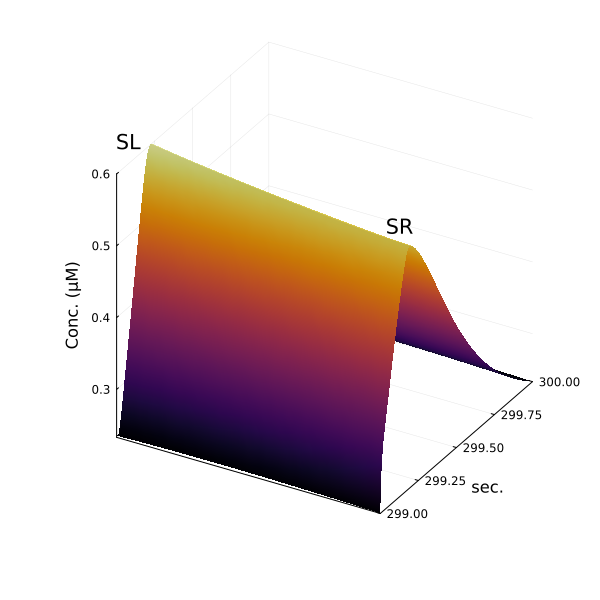
savefig("3d-surface.pdf")
"/home/github/actions-runner-2/_work/camkii-cardiomyocyte-model/camkii-cardiomyocyte-model/.cache/docs/3d-surface.pdf"
2Hz#
callback = build_stim_callbacks(Istim, stimend; period=1 / 2 * second, starttime=stimstart)
@time sol2 = solve(prob, alg; callback)
2.650460 seconds (125.96 k allocations: 35.199 MiB)
retcode: Success
Interpolation: 3rd order Hermite
t: 14163-element Vector{Float64}:
0.0
0.019333123284849308
0.09098129546757994
0.20735582943983855
0.45611910276862533
1.0472562928406122
2.0342667657223696
3.451776641148454
6.733792423912806
12.450117081023194
⋮
403252.8064076104
412782.91906571155
423257.62396415835
435362.5046771487
447467.38539013907
461423.863562818
478496.3270349015
495568.790506985
500000.0
u: 14163-element Vector{Vector{Float64}}:
[150952.75035000002, 13838.37602, -68.79268, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113, 0.12113 … 0.26081, 0.00977, 0.00188, 0.09243, 0.22156, 0.966, 0.00702, 830.0, 830.0, 0.0026]
[150952.7504077194, 13838.375711519551, -68.79732946186226, 0.1211319185255175, 0.1211301421535992, 0.12113000811850358, 0.12113000037367234, 0.12113000001389383, 0.12113000000038378, 0.12113000000000405 … 0.2608055799112056, 0.009769882842069916, 0.001879826176081169, 0.09242766669618695, 0.2215639938807744, 0.9660018276402699, 0.007020208561323646, 829.9999654017583, 829.9994975880693, 0.0025985659590295374]
[150952.75062244057, 13838.374513299192, -68.81607005265552, 0.12115248970210647, 0.12113624253259858, 0.12113143375871602, 0.12113027926811751, 0.12113004704056851, 0.12113000693481112, 0.12113000089551408 … 0.26078941009382306, 0.009769459049732313, 0.0018789042446514422, 0.09241901973901424, 0.2215787945110049, 0.9660085993318799, 0.00702008986027517, 829.9998348635061, 829.9976422525165, 0.002593310524681686]
[150952.7509740238, 13838.372555328333, -68.84681913285226, 0.12118951551963696, 0.12115723910142134, 0.12114109135582982, 0.12113403405679991, 0.12113131833063846, 0.12113038966346883, 0.12113010483012127 … 0.2607638707001999, 0.009768805322556195, 0.0018766436937193197, 0.09240497563401104, 0.22160283236040595, 0.9660195931792828, 0.007017234889558555, 829.9996133960318, 829.9946506157202, 0.0025849478335103777]
[150952.7517373371, 13838.368384399068, -68.91207316777846, 0.12125418995384041, 0.12120713813483534, 0.12117519662647316, 0.12115494411090462, 0.12114295934422187, 0.12113633873395535, 0.12113292110288301 … 0.2607122383588733, 0.00976754294689657, 0.0018699196262940568, 0.0923749578279845, 0.2216542075240971, 0.9660430731013478, 0.0070030143052343926, 829.9990964774663, 829.9883445519497, 0.00256770780104998]
[150952.75361533565, 13838.358559292059, -69.06429960994137, 0.12136450208851983, 0.1213063255235844, 0.12125925929399752, 0.12122229800810434, 0.12119414515426227, 0.1211733598588045, 0.12115849269665879 … 0.26060507831375035, 0.009765228548607825, 0.001850533769446942, 0.09230364339951613, 0.22177624785138977, 0.9660987611411453, 0.006946470397388757, 829.9976227528975, 829.9738229154025, 0.0025298083713907005]
[150952.75694915713, 13838.34240329337, -69.31024285744343, 0.1214919651325424, 0.12142927821043338, 0.12137426379919636, 0.12132672249013034, 0.12128628091507473, 0.12125242557826561, 0.12122454121844703 … 0.26047114911179464, 0.009763403076884485, 0.0018178174419014683, 0.09218462776986637, 0.2219798989175973, 0.9661914345949096, 0.0068337541728754475, 829.9944193668179, 829.9509077855928, 0.0024748079782158957]
[150952.76215620188, 13838.319723120678, -69.64637448767432, 0.12162015426876954, 0.12155743299711902, 0.12149988983034497, 0.1214475947100359, 0.12140052118634262, 0.12135855488207907, 0.12132150441596071 … 0.2603648729749554, 0.009765046552800477, 0.001773815690753224, 0.09201383579531924, 0.22227216398019892, 0.9663239343003682, 0.006675512062656749, 829.9883533251192, 829.9205247545176, 0.0024106893964811324]
[150952.77598869594, 13838.2694938995, -70.35063788657428, 0.12180447948880577, 0.12174768443532356, 0.12169340184085964, 0.12164180995533558, 0.12159304920072024, 0.12154722283384184, 0.12150439833798556 … 0.26041507667541997, 0.009786689200123853, 0.0016855899094922325, 0.09161908329520764, 0.22294828828976576, 0.9666286957812559, 0.006355039713677859, 829.9689941817725, 829.8589858006806, 0.002310655727734339]
[150952.80529264064, 13838.18882346682, -71.35304509818071, 0.12196378364408175, 0.12191937753328333, 0.12187579038290348, 0.1218331857334074, 0.12179171693004406, 0.12175152667167365, 0.12171274673876366 … 0.26104417804603025, 0.009873892913308327, 0.0015688568862426045, 0.09093422465365385, 0.22412560303273427, 0.9671554512230358, 0.005926150085676071, 829.923524981244, 829.7704141396295, 0.002228995040224517]
⋮
[151329.73678923384, 13463.639830820652, -70.16237636695821, 0.13519778379973146, 0.13519809955808137, 0.1351984159261927, 0.13519873297816615, 0.13519905079109867, 0.13519936944523614, 0.13519968902413584 … 0.0011632495547059388, 0.0015991246689223185, 0.0024753321190406027, 0.0015704594566432872, 0.9997345986850064, 0.9997325765508916, 0.006401536598238153, 783.5706100264493, 783.2335456223694, 0.0019114696198640015]
[151381.23645949643, 13412.288684613293, -70.06630769034012, 0.13454769203651706, 0.1345479974090137, 0.13454830348748906, 0.1345486103459281, 0.13454891806130756, 0.13454922671374847, 0.13454953638667824 … 0.0011806758442164003, 0.0016105830832264189, 0.0024930649558123547, 0.0015922867934417973, 0.9997289833818177, 0.9997269806387864, 0.0064434881853996295, 782.6921656330787, 782.3652785609543, 0.0018559861797096816]
[151435.35010948055, 13358.332495903609, -69.9640696394178, 0.13387422033556315, 0.133874514412593, 0.13387480928394224, 0.13387510502315123, 0.13387540170673387, 0.13387569941432997, 0.13387599822886562 … 0.0011995128908701429, 0.0016228666615522296, 0.002512074324108596, 0.0016158415907307397, 0.9997228759655545, 0.9997208967515301, 0.006488433583871601, 781.7426554921773, 781.4261787177528, 0.0017991858469342766]
[151494.7736102139, 13299.083470563586, -69.85023064464455, 0.13314619958237173, 0.1331464808296654, 0.13314676295382977, 0.13314704602754976, 0.13314733012644897, 0.13314761532923952, 0.1331479017178813 … 0.0012208313551400297, 0.00163665195126196, 0.0025334080706054625, 0.001642469297394791, 0.9997159113620444, 0.9997139634414584, 0.006538841664683359, 780.6694749774759, 780.3640957242394, 0.001738578679832934]
[151551.04143769512, 13242.982305626698, -69.74087130994276, 0.13246809162642303, 0.13246836026157868, 0.13246862983713323, 0.13246890042458132, 0.13246917209830814, 0.13246944493573679, 0.132469719017485 … 0.0012416666191177777, 0.0016500054458299233, 0.00255407336765699, 0.0016684544490971436, 0.9997090568751028, 0.9997071390528248, 0.006587639980610353, 779.6244064032337, 779.3292147839669, 0.0016828788058262018]
[151612.22778821783, 13181.978662174575, -69.62018325293539, 0.13174335338581206, 0.13174360786396697, 0.13174386333520424, 0.13174411986930085, 0.13174437753885535, 0.13174463641943124, 0.13174489658970995 … 0.0012650759161119511, 0.001664866164793696, 0.0025770708216702313, 0.0016975971965945955, 0.999701297436267, 0.999699419469964, 0.006641908415666428, 778.4573206864591, 778.1728546367142, 0.001624167445903346]
[151682.02374670643, 13112.39281500162, -69.48017476805369, 0.13093303536225412, 0.1309332731036888, 0.13093351187917793, 0.13093375175604346, 0.130933992804329, 0.13093423509693877, 0.1309344787097851 … 0.0012927869694995139, 0.0016822714393580334, 0.0026040058676421005, 0.0017320283400833886, 0.999692034705106, 0.999690208786094, 0.006705419682529862, 777.0886734375172, 776.8159951664793, 0.0015595490771180638]
[151746.66233734848, 13047.950238293064, -69.34821396043414, 0.130198460833353, 0.13019868256585695, 0.13019890535054332, 0.1301991292519654, 0.130199354337286, 0.1301995806764107, 0.13019980834212894 … 0.0013194623376822513, 0.0016988403880178143, 0.0026296463463495517, 0.0017651047130269753, 0.9996830392302856, 0.9996812693521222, 0.006765829406155109, 775.7874674618943, 775.5252842341403, 0.0015019225050593083]
[151762.6446088316, 13032.016623562431, -69.31523435344212, 0.13001921661951066, 0.1300194343218719, 0.13001965307814134, 0.13001987295212936, 0.13002009401022563, 0.13002031632153044, 0.13002053995799448 … 0.0013262160662111627, 0.001703006764205151, 0.0026360940080447696, 0.0017734683214279871, 0.9996807506215253, 0.9996789946620139, 0.006781012883966346, 775.4609759601381, 775.2013254250732, 0.0014880014404269847]
plot(sol2, idxs=(sys.t / 1000, sys.vm), title="Action potential", ylabel="mV", xlabel="Time (s)", label=false)
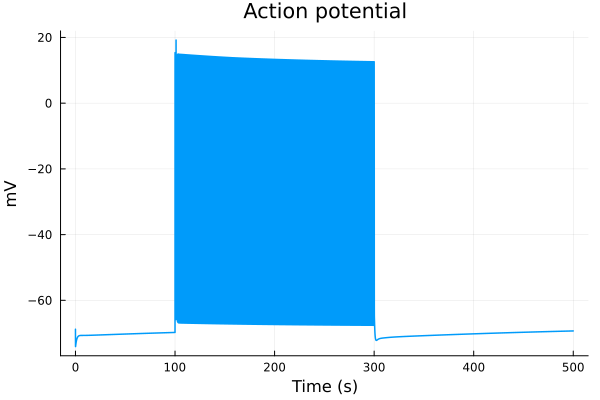
plot(sol2, idxs=(sys.t / 1000 - 299, sys.vm), title="Action potential", tspan=(299second, 300second), ylabel="mV", xlabel="Time (s)", label=false)
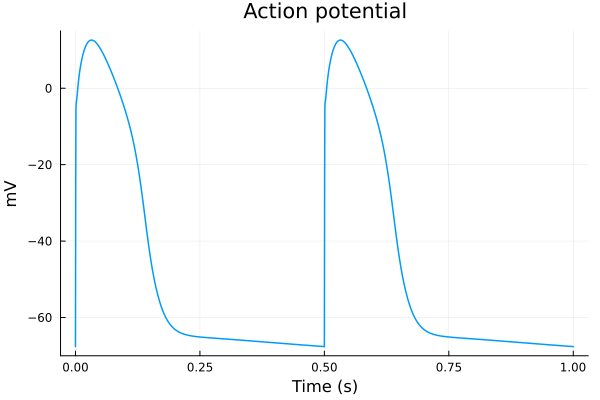
plot(sol2, idxs=(sys.t / 1000 - 299, [sys.Cai_sub_SR, sys.Cai_sub_SL, sys.Cai_mean]), tspan=(299second, 300second), title="Calcium transient", ylabel="Concentration (μM)", xlabel="Time (s)", label=["CaSSR" "CaSL" "CaAvg"])
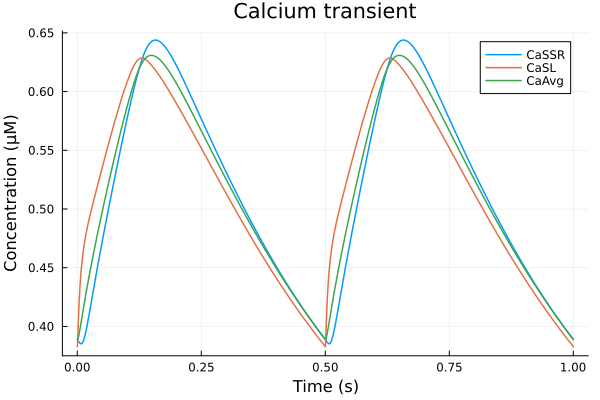
plot(sol2, idxs=(sys.t / 1000, sys.CaMKAct * 100), title="Active CaMKII", ylabel="Active CaMKII (%)", xlabel="Time (s)", label=false)
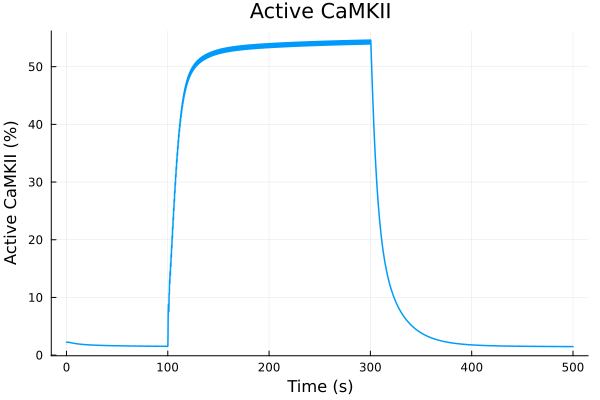
Comparing 1 and 2 Hz pacing#
idxs = (sys.t / 1000 - 299, sys.vm)
plot(sol, idxs=idxs, title="Action potential", lab="1Hz", tspan=(299second, 300second))
plot!(sol2, idxs=idxs, lab="2Hz", tspan=(299second, 300second), xlabel="Time (s)", ylabel="Voltage (mV)")
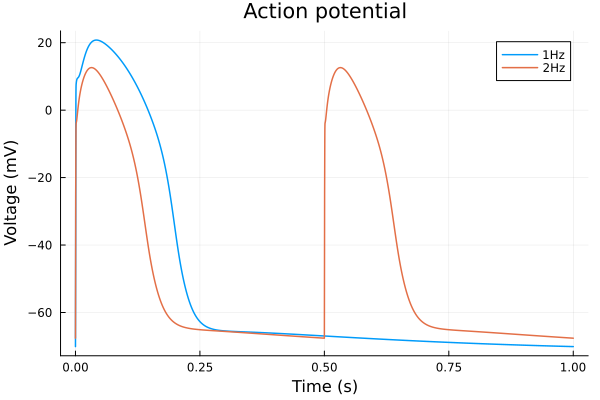
savefig("bcl-ap.pdf")
"/home/github/actions-runner-2/_work/camkii-cardiomyocyte-model/camkii-cardiomyocyte-model/.cache/docs/bcl-ap.pdf"
idxs = (sys.t / 1000 - 299, sys.Cai_mean)
plot(sol, idxs=idxs, title="Calcium transient", lab="1Hz", tspan=(299second, 300second))
plot!(sol2, idxs=idxs, lab="2Hz", tspan=(299second, 300second), xlabel="Time (s)", ylabel="Concentration (μM)")
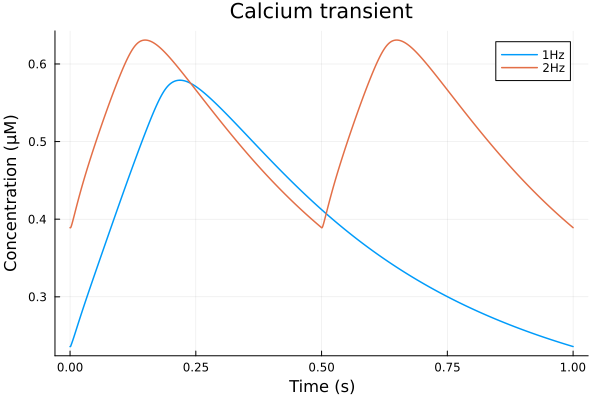
savefig("bcl-cat.pdf")
"/home/github/actions-runner-2/_work/camkii-cardiomyocyte-model/camkii-cardiomyocyte-model/.cache/docs/bcl-cat.pdf"
idxs = (sys.t / 1000, sys.CaMKAct * 100)
plot(sol, idxs=idxs, title="CaMKII", lab="1Hz")
plot!(sol2, idxs=idxs, lab="2Hz", xlabel="Time (s)", ylabel="Active fraction (%)")
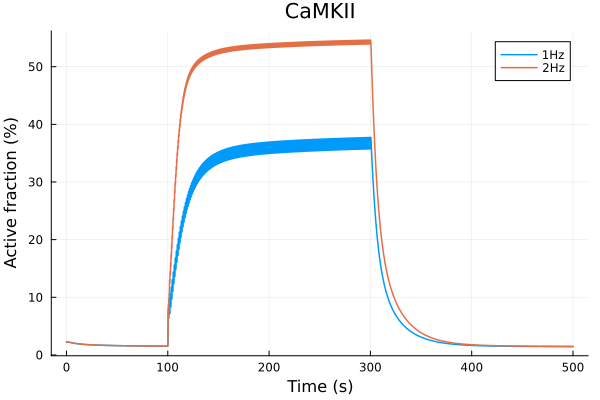
savefig("bcl-camkact.pdf")
"/home/github/actions-runner-2/_work/camkii-cardiomyocyte-model/camkii-cardiomyocyte-model/.cache/docs/bcl-camkact.pdf"
This notebook was generated using Literate.jl.